top of page
University Projects
C++ OpenGL project

A Work In Progress
C++ / OpenGL Project
I have embarked upon this project as a way to keep my skills sharp and to learn new concepts in the world of C++, OpenGL and design patterns.
The current feature set for the project includes:
-
Function delegates built using templates. These delegates allow regular C functions, C++ member functions and C++ lambda functions to be dynamically bound and invoked.
-
Custom dynamic arrays that are capable of dynamically changing size, with space being allocated using a custom allocator.
-
A custom built math library for handling matrices, quaternions and vectors, amongst other useful functionalities.
-
An input manager that uses the custom delegates for various input callbacks, namely when the mouse moves and when keys are held down.
-
Basic texture loading support for BMP, TGA and DDS textures, with flip support for a compressed DDS texture if needed.
This is just some of the functionality that I've managed to implement with more nifty features to follow as I continue to work on and expand the project.
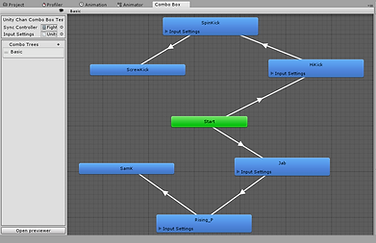
The Combo Box
May 2017
The Combo Box was my final year project. The task which I set myself was to create an editor for the Unity Engine. This editor would allow for the creation of melee combo trees, similar to those seen in fighting games like Devil May Cry or Prince of Persia.
Features for the project included:
-
C++ back-end. This took the form of a DLL and it was the home of the runtime logic.
-
C# front-end. This was used to communicate with the back-end and also to leverage Unity's serialisation system.
-
C# editor. This was used to create the combo trees and necessary assets for the combos and moves to work.
The whole project was then packaged up with the necessary report that described the process and talked about certain features of the project in greater depth.
Overall, this was a fun project that worked to enhance my understanding of C++ and C#, and it neatly demonstrated how to get the two to work together.
C++ game framework
C++ Game Project
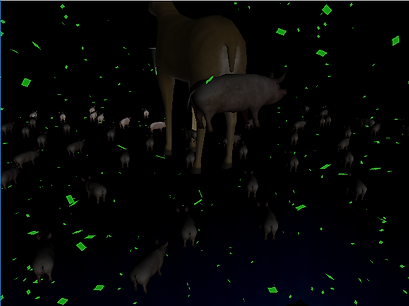
Jan 2017
This was an interactive application using Directx11 and no smart pointers. This project was done entirely in C++ and, whilst not a proper game, it still had some cool under-the- hood gimmicks that I was pleased with.
The project featured:
-
Custom shaders that provided simple lighting effects and four distinct sky-boxes.
-
A micro game engine that managed all the game objects that were in use.
-
Instance rendering that was used to render both swirly 'particles' and 'miniature pig' Boids.
-
A mesh loader that could load .obj files and their corresponding .mtl material.
-
Input handling using the DirectX8 input API.
The project was fun to work on and with all the additional gimmicks that I threw in, it also proved great for my understanding of programming in C++.
Pirate Panic
Pirate Panic
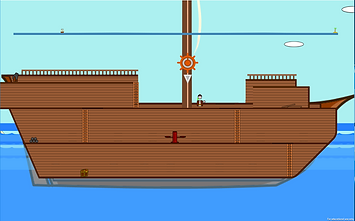
Jan 2017
Pirate Panic was a game developed by Directional Games, a spoof company that was set up as part of a university module that had us go through the process of creating a product and taking it to market.
The game was built in Unity3D and was a 2D co-operative multiplayer game featuring piratical adventures on the high seas. The goal of the game was to reach the end of the level with as much gold as possible.
My role in the development of the game was that of programmer and designer. Some of the features I worked on included:
-
The character controller, for moving the character and defining how they should interact with certain objects in the scene.
-
The mechanics for firing the cannon, giving it more kick than necessary when fired.
-
The implementation for the sails, cannon and bilge pump objects in the scene.
-
I wrote the necessary code to handle scoring and moving the ship, as the ship doesn't actually move.
-
Bug fixing and refactoring as we did a lot of iterating, which games rely on.
Needless to say, although tricky in places, the project was a fun one to work on. After the project was complete, we were asked to showcase the game at Plymouth University's 2017 Science and Engineering Faculty Showcase.
Mobile Infinite Runner
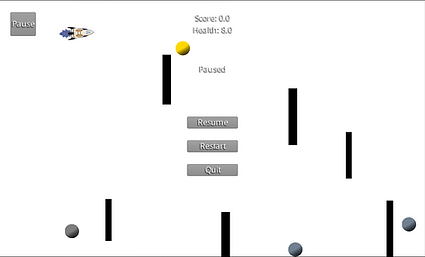
May 2016
This was a university project requiring group co-operation yet again. We were plunged unwitting into the dark and mysterious world of mobile software development. The task presented to us was the creation of a mobile application that could be either a game or a regular app. Our foray into the depths saw us returning with our completed project, the unofficially dubbed "Mobile Infinite Runner". The game was built without Unity, a first for us, being built instead with an alternative framework libgdx.
I was the team member tasked to handle most of the gameplay related features, including:
-
Implementing a pooling system to reuse objects in the game, namely the collectables and obstacles.
-
Implement the collectables and obstacles, write the code to make them work.
-
Implement the standard issue movement controls for the character, making use of mouse movement for computer testing.
-
Create the necessary functionality to load the scenes we had made, all generated in code.
-
Integrate the mandatory networking component that another team member had created, an online high-score leader board.
Overall, the project provided me with the opportunity to learn about mobile development, new design patterns and new gaming frameworks.
University Swipe Card System
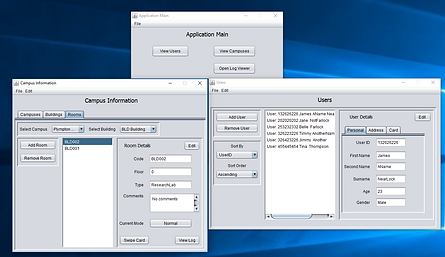
Jan 2016
This project was created as part of a module specifically about software design patterns. The assignment brief was to create a system that would be used to control swipe card access to rooms, and to hold information about the various cardholders. The system was built using Java, and consisted of two parts: a core data structure and a display.
My implementation of the system featured:
-
Heavy usage of interfaces. Most of the core classes used interfaces to promote easy expansion of core systems.
-
A variety of design patterns, ranging from the humble Singleton pattern to the more esoteric Builder pattern. These patterns were used in the core system and the display.
-
Undo and redo functionality for most of the system's features, courtesy of the Command pattern.
-
Binary saving and loading, allowing the data to persist between sessions. There was also text saving for log files, used to monitor various aspects of the rooms.
-
Swipe card simulation allowing the user of the system to simulate a swipe for a room. Useful to check if credentials are set up correctly or for testing the system.
I invested a lot of time in this project and was pleased with the result. I provided everything the core spec wanted and more, giving me valuable knowledge to take forward and use on other systems.
The Disappearance At Kraden Manor
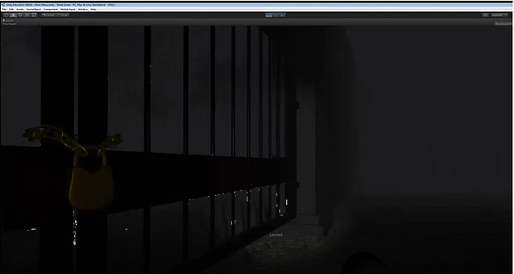
Jan 2016
This was a project I created in my second year. The aim of the project was to produce a simple interactive experience using primarily custom built assets. I decided to create a horror-style experience making use of the Unity game engine, with my main focus being on the design of the experience.
The experience included various features like:
-
A functioning flashlight to light up the dark murky areas. It was also used to highlight objects that the player could interact with.
-
Custom built sounds, borrowed from the internet and modified, the sounds were used to help set the tone for the experience and to try to build tension.
-
Custom built models. The models were built in 3ds Max and used in the level. Models included the flashlight, padlock and chain, and the imposing wrought iron gates.
-
Custom textures. As with the sound, they were borrowed from the internet and modified accordingly. I also UV mapped them onto the models.
The project was an interesting one and provided me with my first stab at trying to create an interactive experience using self-made assets. This really highlighted aspects of the production process that I might've otherwise glossed over and proved to be a valuable learning experience.
XNA Item Collector
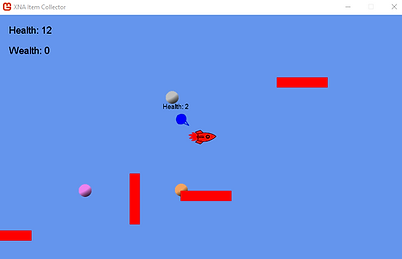
April 2015
XNA Item Collector was a game I built as part of a first year university module. For this project, I built an Item collection game using the XNA framework and MonoGame. I spent a lot of time honing my object oriented skills, with the game's engine making use of inheritance to create reusable functionality.
Features include:
-
Reusable object hierarchies being used to provide functionality for the characters and managers.
-
Two enemy types that used inheritance to control how they seek and destroy.
-
Custom text box classes to help with displaying text on screen.
-
Managers that were responsible for creating and maintaining the enemies, items and obstacles.
-
Randomisation of several in game elements.
This was one of my favourite first year projects. It provided me with a test bed for new object oriented concepts and it came with its own set of challenges that I enjoyed solving.
Micro Racer
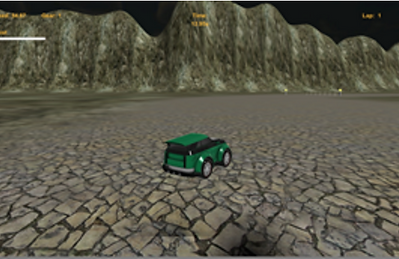
April 2015
Micro racer was the second game that I created during my time at Plymouth University. The game was built in Unity3D and was a single player racing game featuring a child's toy cars. The project assets were provided by the tutors, allowing us to focus on enhancing the experience whilst growing our own understanding of the systems that Unity provided.
The game featured:
-
A boost mechanic. The boost would maximise the speed of the car for a limited time allowing the player, depending on their skill, to either speed on the straights or speed off the map.
-
A drift mechanic. This tool was designed to let players negotiate the turns with ease whilst maintaining speed.
-
A persistent leader board. This board would persist between scenes and was displayed at the end of every race. I also wrote the algorithm to sort the entries.
-
Custom built track. The terrain was built with Unity's terrain tools and the checkpoint system was modified to place lights on the checkpoints, letting you know which one was next.
I don't often play racing games, so it was an interesting opportunity to make one. Nevertheless, I learnt some useful things about Unity and the various challenges you stumble across when building such games.
Zombie Hunter
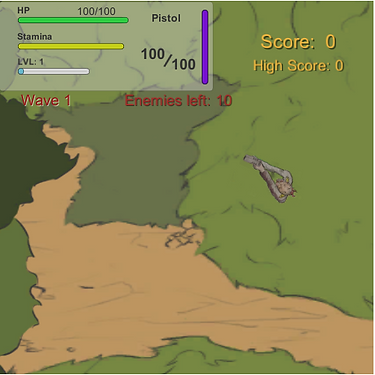
January 2015
Zombie Hunter was the very first game that I developed and signalled my first step along the path of game development. The game was a top down shooter that was built within the Unity engine, and it was one of my first year assignments.
This game provided me with the opportunity to see what the game making process was all about and I used that opportunity to the fullest.
The final submitted version included:
-
A custom built inventory system. The game had several weapons to choose from and you could cycle between any of the weapons you had in your inventory.
-
Enemy waves. Enemies would spawn randomly around the arena and I provided a handy counter so you could tell how many enemies were left. Randomised enemy movement was also incorporated.
-
Extensive use of the, then new, Unity UI tools allowing for the creation of the main menu and player HUD.
-
Out-sourced artwork. I tapped an artistic friend for the character artwork.
-
Item drops. The zombies dropped ammo, med kits and, during certain waves, they would drop new weapons.
-
Multiple enemy types. Some enemies had different numbers defining their behaviour whilst some, like the Leaper, had unique abilities to keep the player on their toes.
-
Shooting zombies. It wouldn't be a zombie shooter without the ability to shoot zombies, and the game might as well have been called Zombie Fodder instead.
Overall, it was one of my favourite first year projects to work on. The project was an invaluable test bed to test out new Unity systems and programming concepts.
bottom of page